Client-side storage
Modern web browsers support a number of ways for websites to store data on the user's computer — with the user's permission — then retrieve it when necessary. This lets you persist data for long-term storage, save sites or documents for offline use, retain user-specific settings for your site, and more. This article explains the very basics of how these work.
Client-side storage?
Elsewhere in the MDN learning area, we talked about the difference between static sites and dynamic sites. Most major modern websites are dynamic — they store data on the server using some kind of database (server-side storage), then run server-side code to retrieve needed data, insert it into static page templates, and serve the resulting HTML to the client to be displayed by the user's browser.
Client-side storage works on similar principles, but has different uses. It consists of JavaScript APIs that allow you to store data on the client (i.e. on the user's machine) and then retrieve it when needed. This has many distinct uses, such as:
- Personalizing site preferences (e.g. showing a user's choice of custom widgets, color scheme, or font size).
- Persisting previous site activity (e.g. storing the contents of a shopping cart from a previous session, remembering if a user was previously logged in).
- Saving data and assets locally so a site will be quicker (and potentially less expensive) to download, or be usable without a network connection.
- Saving web application generated documents locally for use offline
Often client-side and server-side storage are used together. For example, you could download a batch of music files (perhaps used by a web game or music player application), store them inside a client-side database, and play them as needed. The user would only have to download the music files once — on subsequent visits they would be retrieved from the database instead.
Old school: Cookies
The concept of client-side storage has been around for a long time. Since the early days of the web, sites have used cookies to store information to personalize user experience on websites. They're the earliest form of client-side storage commonly used on the web.
These days, there are easier mechanisms available for storing client-side data, therefore we won't be teaching you how to use cookies in this article. However, this does not mean cookies are completely useless on the modern-day web — they are still used commonly to store data related to user personalization and state, e.g. session IDs and access tokens. For more information on cookies see our Using HTTP cookies article.
New school: Web Storage and IndexedDB
The "easier" features we mentioned above are as follows:
- The Web Storage API provides a mechanism for storing and retrieving smaller, data items consisting of a name and a corresponding value. This is useful when you just need to store some simple data, like the user's name, whether they are logged in, what color to use for the background of the screen, etc.
- The IndexedDB API provides the browser with a complete database system for storing complex data. This can be used for things from complete sets of customer records to even complex data types like audio or video files.
You'll learn more about these APIs below.
The Cache API
The Cache API is designed for storing HTTP responses to specific requests, and is very useful for doing things like storing website assets offline so the site can subsequently be used without a network connection. Cache is usually used in combination with the Service Worker API, although it doesn't have to be.
The use of Cache and Service Workers is an advanced topic, and we won't be covering it in great detail in this article, although we will show an example in the Offline asset storage section below.
Storing simple data — web storage
The Web Storage API is very easy to use — you store simple name/value pairs of data (limited to strings, numbers, etc.) and retrieve these values when needed.
Basic syntax
Let's show you how:
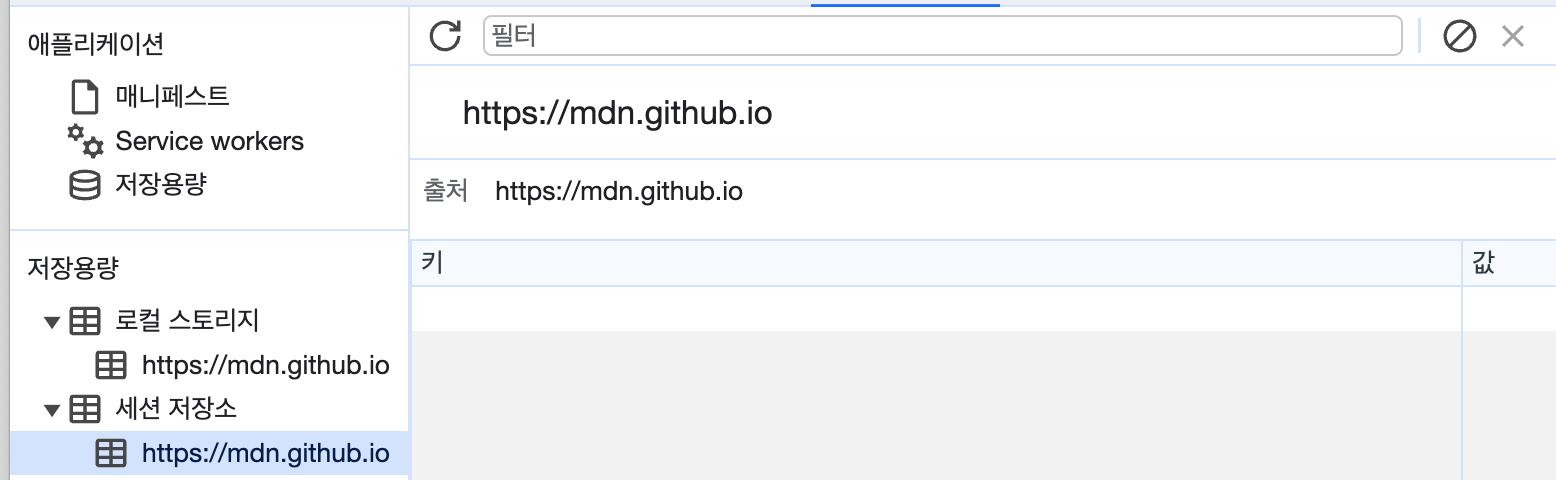
- First, go to our web storage blank template on GitHub (open this in a new tab).
- Open the JavaScript console of your browser's developer tools.
- All of your web storage data is contained within two object-like structures inside the browser: sessionStorage and localStorage. The first one persists data for as long as the browser is open (the data is lost when the browser is closed) and the second one persists data even after the browser is closed and then opened again. We'll use the second one in this article as it is generally more useful. The Storage.setItem() method allows you to save a data item in storage — it takes two parameters: the name of the item, and its value. Try typing this into your JavaScript console (change the value to your own name, if you wish!):
localStorage.setItem("name", "Chris");
- The Storage.getItem() method takes one parameter — the name of a data item you want to retrieve — and returns the item's value. Now type these lines into your JavaScript console:
let myName = localStorage.getItem("name"); myName;
- The Storage.removeItem() method takes one parameter — the name of a data item you want to remove — and removes that item out of web storage. Type the following lines into your JavaScript console:
localStorage.removeItem("name"); myName = localStorage.getItem("name"); myName;

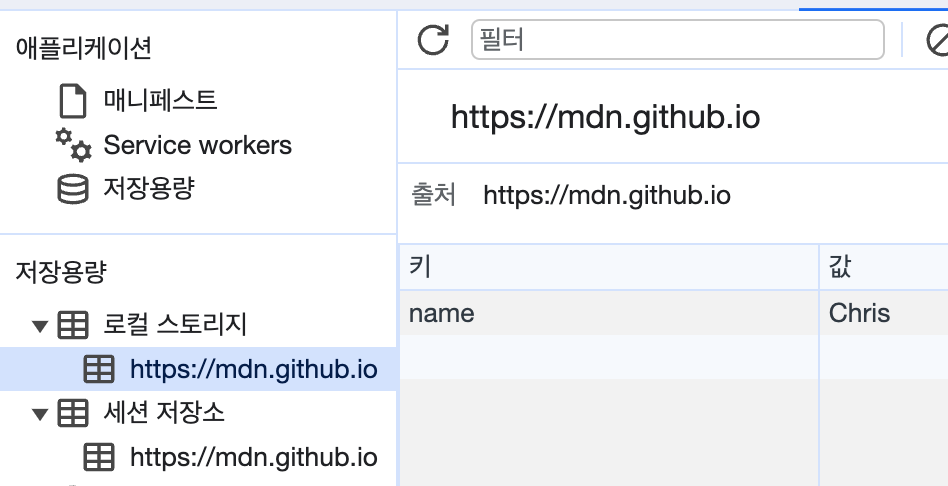
The data persists!
One key feature of web storage is that the data persists between page loads (and even when the browser is shut down, in the case of localStorage). Let's look at this in action.
- Open our web storage blank template again, but this time in a different browser to the one you've got this tutorial open in! This will make it easier to deal with.
- Type these lines into the browser's JavaScript console:
localStorage.setItem("name", "Chris"); let myName = localStorage.getItem("name"); myName;
- Now close down the browser and open it up again.
- Enter the following lines again:
let myName = localStorage.getItem("name"); myName;
로컬 스토리지는 브라우저를 껐다 켜도 데이터가 저장되어 있다.
There is a separate data store for each domain (each separate web address loaded in the browser). You will see that if you load two websites (say google.com and amazon.com) and try storing an item on one website, it won't be available to the other website.
This makes sense — you can imagine the security issues that would arise if websites could see each other's data!
A more involved example
Let's apply this new-found knowledge by writing a working example to give you an idea of how web storage can be used. Our example will allow you to enter a name, after which the page will update to give you a personalized greeting. This state will also persist across page/browser reloads, because the name is stored in web storage.
You can find the example HTML at personal-greeting.html — this contains a website with a header, content, and footer, and a form for entering your name.
Let's build up the example, so you can understand how it works.
- First, make a local copy of our personal-greeting.html file in a new directory on your computer.
- Next, note how our HTML references a JavaScript file called index.js, with a line like <script src="index.js" defer></script>. We need to create this and write our JavaScript code into it. Create an index.js file in the same directory as your HTML file.
- We'll start off by creating references to all the HTML features we need to manipulate in this example — we'll create them all as constants, as these references do not need to change in the lifecycle of the app. Add the following lines to your JavaScript file:
// create needed constants
const rememberDiv = document.querySelector(".remember");
const forgetDiv = document.querySelector(".forget");
const form = document.querySelector("form");
const nameInput = document.querySelector("#entername");
const submitBtn = document.querySelector("#submitname");
const forgetBtn = document.querySelector("#forgetname");
const h1 = document.querySelector("h1");
const personalGreeting = document.querySelector(".personal-greeting");
4. Next up, we need to include a small event listener to stop the form from actually submitting itself when the submit button is pressed, as this is not the behavior we want. Add this snippet below your previous code:
// Stop the form from submitting when a button is pressed
form.addEventListener("submit", (e) => e.preventDefault());
5. Now we need to add an event listener, the handler function of which will run when the "Say hello" button is clicked. The comments explain in detail what each bit does, but in essence here we are taking the name the user has entered into the text input box and saving it in web storage using setItem(), then running a function called nameDisplayCheck() that will handle updating the actual website text. Add this to the bottom of your code:
// run function when the 'Say hello' button is clicked
submitBtn.addEventListener("click", () => {
// store the entered name in web storage
localStorage.setItem("name", nameInput.value);
// run nameDisplayCheck() to sort out displaying the personalized greetings and updating the form display
nameDisplayCheck();
});
6. At this point we also need an event handler to run a function when the "Forget" button is clicked — this is only displayed after the "Say hello" button has been clicked (the two form states toggle back and forth). In this function we remove the name item from web storage using removeItem(), then again run nameDisplayCheck() to update the display. Add this to the bottom:
// run function when the 'Forget' button is clicked
forgetBtn.addEventListener("click", () => {
// Remove the stored name from web storage
localStorage.removeItem("name");
// run nameDisplayCheck() to sort out displaying the generic greeting again and updating the form display
nameDisplayCheck();
});
7. It is now time to define the nameDisplayCheck() function itself. Here we check whether the name item has been stored in web storage by using localStorage.getItem('name') as a conditional test. If the name has been stored, this call will evaluate to true; if not, the call will evaluate to false. If the call evaluates to true, we display a personalized greeting, display the "forget" part of the form, and hide the "Say hello" part of the form. If the call evaluates to false, we display a generic greeting and do the opposite. Again, put the following code at the bottom:\
\\
This is where Service workers and the closely-related Cache API come in.
A service worker is a JavaScript file that is registered against a particular origin (website, or part of a website at a certain domain) when it is accessed by a browser. When registered, it can control pages available at that origin. It does this by sitting between a loaded page and the network and intercepting network requests aimed at that origin.
When it intercepts a request, it can do anything you wish to it (see use case ideas), but the classic example is saving the network responses offline and then providing those in response to a request instead of the responses from the network. In effect, it allows you to make a website work completely offline.
The Cache API is another client-side storage mechanism, with a bit of a difference — it is designed to save HTTP responses, and so works very well with service workers.
'【 개발 이야기 】' 카테고리의 다른 글
[github] organization (0) | 2024.09.05 |
---|---|
[intelliJ] junit 같은 인텔리제이 내 자바 실행기 (0) | 2023.10.24 |
F/E 기초 - API (0) | 2023.10.12 |
F/E 기초 - JSON (2) | 2023.10.11 |
F/E 기초 (3) | 2023.10.06 |